Im trying to write a script to put an indicator on the chart when the RSI levels hit 30. Cannot figure out how to do this. Any suggestions would be great!
Help on for command
Hello
I would like to know the nearest flat SSB (ichimoku) value above closing price. I wrote this but this does not work properly and I don’t know why… any idea?
flatichimokulevel=input(9)
isflat(x) =>
out = true
for i = 0 to flatichimokulevel-1
out := out and (x[i] == x[i+1])
out
lowestFlatSSBabove(p) =>
out=close
ssb=leadingSpan2
for i = 0 to (p+displacement)
ssbi=ssb[i]
out := (ssb[i] <= ssb[i+1] and ssb[i] >= close and isflat(ssbi)) ? ssb[i] : out
out
Recalling a variable
If you’re a novice programmer in Pine, you may have encountered the situation of needing that remember a value during cycles.
The graph draws the bars from left to right and from the beginning (or from a particular time), we can assign a value to a variable (although it will not draw) and keep this reminder over the time.
To remember a value between a bar and the next is needed to copy the previous value of this variable in the current variable.
variable = variable[1]
The first bar not have a previous bar. Then, to assign a first value you must to use the function nz( ) that detects whether a variable is uninitialized (empty) and if so, it assigns the initial value …
variable = nz(variable[1],23)
In this example the initial value is 23. This variable will always have the value 23 because this code copy its value from the previous bar.
If you need to assign other value when a condition occurs then you can use this code…
variable = condition? new_value : nz(variable[1],23)
PineScripts.
The ‘precision’ parameter
The ‘study’ and ‘strategy’ commands have this parameter.
Is the number of digits after the floating point for values on the price axis. Must be a non negative integer. Precision 0 has special rules for formatting very large numbers (like volume, e.g. ‘5482’ will be formatted as ‘5K’). By default, the value is 4.
But remember:
THE PRECISION PARAMETER IS ONLY TO SHOW THE PRICE AXIS IN GRAPHIC BUT DOES NOT AFFECT TO SCRIPT CALCULATIONS.
This means that floating point prices will not are rounded to the decimals depending on the “precision” parameter.
PineScripts.
BLOCKS: user functions, ‘if’ and ‘for’ commands
This is not a reference site for Pine scripting language (for more information visit Reference Manual and PineScript Tutorial)
Some statements needs to use more than one lines of code, this elements works as a block of commands.
The functions can be defined as only one line or have multiple lines too.
The “for” and “if” statements always have more than one line of code (like multiline functions)
The blocks of commands are defined adding a margin (a tabulator or 4 spaces) by each level.
When “if” and “for” statements are combined (or used into a user defined function) must to add tabulators in each level of nest to separate differents blocks of commands.
Example of structure…
commands of script
commands of script
UserFunction(param1,param2)=>
····commands of function
····var1=if close>open
········commands of if block
········commands of if block
········var2=for c=1 to 10 by 1
············commands of for block
············commands of for block
············value for assignation to var2
········commands of if block
········value for assign to var1 when true
····else
········commands of if block
········value to be assigned to var1 when false
····commands of function
····response value of the function
commands of script
commands of script
As you see, the blocks are defined including a tabulator (4 spaces) or some tabulators (4 + 4 + 4 … spaces) nested in base of the number of levels in the blocks.
The block of statements can recognize vars declared outer of the blocks but the variables defined into them are locals (and can not be accessible in the rest of the script)
ABOUT ‘IF’ STATEMENT
Can not have commands after definition in the first line.
The “else” word must be at the same level of margin of the “if” declaration and can not have other commands in the same line.
The variable gets its value from the last tabulated line of the block (or the last tabulated line of each “else” sub-block, depending on the result of the condition)
variable=if [condition]
····commands if true
····commands if true
····return value if true
else
····commands if false
····commands if false
····return value if false
ABOUT ‘FOR’ STATEMENT
The first line only can have the loop configuration.
The variable used for the loop counter can not be mutable.
Can include “break” or “continue” statements to manipulate the loop conditions interactively.
The variable gets its value from the last tabulated line of the block.
variable=for [counter initiation] to [limit] by [incremental value]
····commands of loop
····commands of loop
····continue
····commands of loop
····commands of loop
····break
····commands of loop
····return value
ABOUT USER FUNCTIONS
Remember the functions must be situated in the top of script (always before be called).
You can not declare functions into other functions, this is not allowed.
The definition of user functions can not have other commands before (in the same line).
The compiler understands as a multilinear function when (in the first line) there is nothing after the symbol ‘=>’
The function returns the value in the last tabulated line.
UserFunction(param1,param2)=>
····commands of function
····commands of function
····commands of function
····return value
REAL EXAMPLE
Here you have a simple code mixing all block statements…
//@version=2
study("WWW.PINESCRIPTS.ORG",overlay=false)
myFun(param)=>
v=for f=1 to param by 1
if close[f]>close
break
f
plot(myFun(100), style=columns)
IMPORTANT
If you get used to separate expresions by some lines you must always remember not to add margins with tabulators or spaces in quantity multiple of 4, because the interpreter could be confuse with BLOCKS of instructions and reports error. You can separate a expresion in some lines like this…
// this works fine
a=close>open?
1:
0
plot(a)
…but the compiler will reports error if you add a margin of separation of a tabulator or 4 spaces (or multiple of 4)….
// this results error
a=close>open?
1:
0
plot(a)
Losing bars/candles
When the symbol with we are working does not have enough liquidity, the platform can display graphics deformed with fewer quantity of candles than usual.
This “loss” of bars/candles is because the plotter display a element only if there were movements during the time corresponding to said bar, depending on the timeframe we’re working.
A lowest timeframe (1 minute, 5 minutes …), has more probability to get this problem.
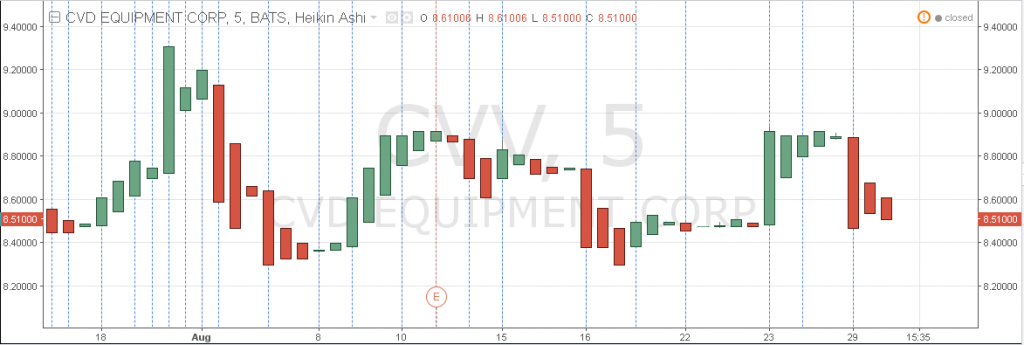
CVD Equipment Corp (CVV) with Heiken Ashi candles at 5 minutes. The lack of liquidity for this share reports a graphic with 1-5 candles by day (instead of 77 with other more liquid share or merchant)
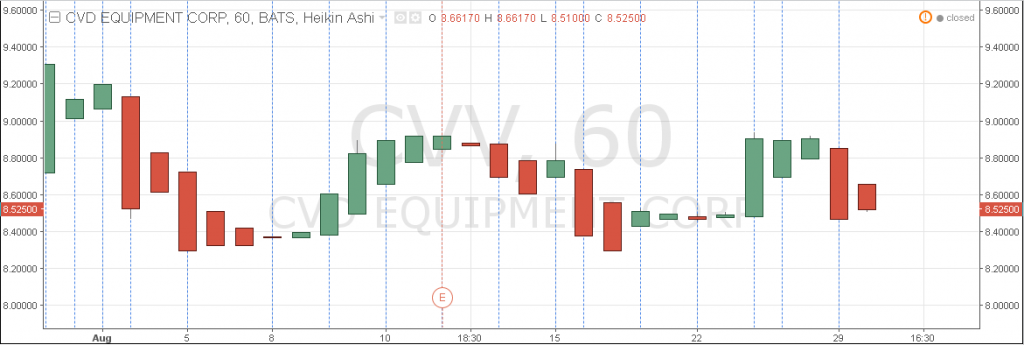
The same company with Heiken Ashi candles at 1 hour. The lack of liquidity for this share reports a graphic with 1-3 candles by day (instead of 6 with other more liquid share/subyacent)
PineScripts.
PineScripts protector. Code encryptor.
The online tool to encrypt pinescript source code is in preparation.
Meanwhile, if you need to encrypt a code contact us at…
Visit our Facebook page
PineScripts.
Not paint in a position
If you want to leave a bar space without painting you can use the na constant.
//@version=2
study("My Script")
x=n%10 // n is the bars counter of the system
r=x==7?na:x // if the value=7 then it not paints
plot(r,style=columns)
This is a counter of bars modulated in 10 (it generates 0-9 values continuosly)
It will paint columns with numbers 0-9 (except the position 7).
Pinescripts.
Charts generation
Before add your script, you must remember that all the elements showed in the chart (bars, candles, indicators…) will be generated from left to right in a only one time.
After the execution of your script (continuing with real time) it will generate the next bars one by one adding to the painted graphic.
Pinescripts.
WHAT IS … //@version=2
You must to include always the comment line at begin of the script …
//@version=2
because it alows to use all the capacityes of the PineScript language and includes the last changes and updates.
PineScripts.
Welcome to PINESCRIPTS.COM
In this website you’ll find tips, tricks, tools, scripts and many information to help you develope robots and trading indicators in Pinescript programming language.
PineScript is a programming language especially dedicated to the creation of financial tools.
PineScripts.